The art of software development often involves striking a delicate balance between creating intricate, powerful applications and maintaining clean, efficient code. To pull off this balancing act, software engineers must first understand cyclomatic complexity in software engineering.
But what is cyclomatic complexity? What can it tell you about a program? How can software programmers use it to drive value and efficiency in their projects?
What is Cyclomatic Complexity?
Cyclomatic complexity is a software metric that quantitatively measures the complexity of a program’s code. It helps developers build an understanding of the intricacy of their programs, enabling them to make decisions about code changes and maintenance. Backed by this deeper understanding of their program’s structure, programmers can measure the code’s intricacy with more accuracy and understand the implications that intricacy may have on the maintainability, reliability, and overall quality of a piece of software.
First developed by Thomas J. McCabe Sr. back in 1976, cyclomatic complexity is based in graph theory and the evaluation of control flow graphs. The underlying principle behind his work was to provide programmers with an easily understood way to identify potential areas of complexity in a program. He accomplished this by creating a metric that examined the various routes through which code execution can take place. This analysis would then provide insights into the code’s structural intricacies, revealing how challenging it may be to test and debug programs since more complex code would naturally require more extensive testing procedures.
It is important to note that the sole determinant of code efficiency cannot only be cyclomatic complexity, meaning other factors must also be taken into account. These factors may include code readability, code complexity, and cognitive complexity. However, cyclomatic complexity is a valuable tool for assessing software quality and provides a useful starting point when identifying areas for improvement or refactoring.
While measuring cyclomatic complexity can help engineers understand the technical intricacy of their code, it is important for leaders not to get too caught up in tactical metrics. In order to contribute to the business effectively, engineering leaders must strive to influence product strategies and define the trajectory of what might lie ahead. Today, software engineering is not confined to the technical precincts but embraces business aspects. By merging core engineering prowess with business acumen, leaders can construct software that not only stands the test of time but also provides quantifiable business impact.
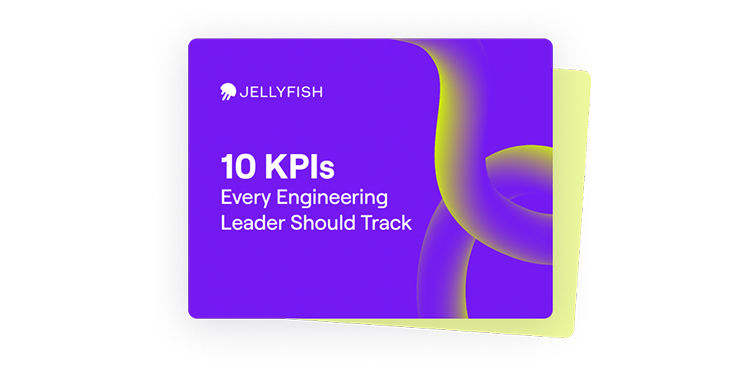
How To Calculate Cyclomatic Complexity
Now that we understand the overall purpose of this important metric, let’s find out how to calculate cyclomatic complexity. Calculate the number of linearly independent paths through a program’s source code, which corresponds to the number of decision points within the code plus one. Essentially, this formula helps to gauge how much branching occurs within a program, as each branch introduces more potential paths for execution.
To effectively apply this formula in software testing, it’s essential first to represent your source code as a control flow graph (CFG). A CFG is a directed graph where each node represents a basic block or sequence of non-branching statements and edges signify control flow between those blocks. Once you have created the CFG for your source code, you can start calculating cyclomatic complexity using any of the three methods we will discuss.
The key components of a program’s CFG include:
- Nodes: Individual commands or statements.
- Edges: Connect nodes.
- Connected components: Isolated sections of the graph.
When calculating cyclomatic complexity, you can use 3 different approaches:
- Basic cyclomatic complexity formula: Cyclomatic Complexity = E – N + 2P, where E corresponds to edges, N to nodes, and P to connected components.
- Counting decision points: Cyclomatic Complexity = Number of decision points + 1.
- Summing up predicate nodes: Cyclomatic Complexity = Sum of all predicate nodes + 1.
In each approach, you will calculate an integer value that represents the number of unique pathways through your code. This value indicates not only how difficult it might be for developers to understand but also impacts testers’ ability to ensure optimal performance from their application or system properly. Higher values suggest greater intricacy and reduced comprehensibility while lower numbers imply a more straightforward, easy-to-follow structure.
For example, consider this very straightforward function:
def simple_function(x):
if x > 0:
print(“X is positive”)
else:
print(“X is not positive”)
In this case:
- E = 2 (number of edges)
- N = 3 (number of nodes)
- P = 1 (single connected component)
Using the formula, the cyclomatic complexity is: CC = 2 – 3 + 2*1 = 1
So, the cyclomatic complexity of this function is 1, indicating very low complexity.
By understanding how to calculate cyclomatic complexity, developers and software testers can better manage code quality and ensure that their testing efforts focus on the most critical areas of the program. This increased focus will result in more robust and efficient software with fewer defects. Having a good grasp of cyclomatic complexity calculation also allows teams to allocate resources more effectively and deliver higher-quality products to their end-users.
Cyclomatic Complexity Benefits And Applications
Understanding the most useful cyclomatic complexity benefits and applications can help developers make the most of this valuable metric. Using cyclomatic complexity in software testing clearly identifies problematic pieces of code and highlights where improvements can be made, enabling testers to review and optimize their code with greater efficiency, improve overall software quality, and reduce maintenance costs.
One of the primary benefits of cyclomatic complexity is its ability to shed light on the level of effort required for testing and debugging a program. Quantifying the number of possible paths in a given segment of code using cyclomatic complexity allows developers to prioritize areas that pose greater risk or difficulty during development. This way, they can allocate resources during the testing process more efficiently, ensuring that critical components of the project are thoroughly examined while less complex elements still receive adequate attention.
Another advantage offered by the use of cyclomatic complexity lies in its potential for improving overall software quality by identifying sections of code with high complexity values. High cyclomatic complexity often indicates convoluted logic, excessive branching, or overly intricate nested structures, which can result in reduced readability and maintainability. Such code segments are also more prone to defects due to their inherent intricacy. Developers can target these areas for refactoring or simplification to enhance the clarity and consistency of software while minimizing potential pitfalls.
Quality metrics in software engineering are necessary for evaluating various aspects of a program’s performance and stability. To create a comprehensive picture of a given program, developers cannot focus solely on cyclomatic complexity, but should instead examine a combination of important metrics. When evaluated alongside other metrics such as lines of code (LOC), defect density, or software maintainability index (SMI), cyclomatic complexity helps stakeholders make informed decisions about the development of the program.
Cyclomatic complexity has several practical applications within the testing phases of software engineering such as test case generation, white-box testing techniques (where internal structures are known), or coverage-based test metrics. The metric identifies the different execution paths within a program, enabling testers to create test cases that ensure comprehensive coverage and validation of the software’s logic. This leads to improved reliability and predictability of the final product while reducing the likelihood of post-deployment issues.
For software developers, testers, and project managers seeking to optimize code quality and performance, maximizing the benefits of cyclomatic complexity is vital. Incorporating this metric into a suite of other quality metrics empowers better, more strategic choices regarding both development priorities and resource allocation.
How To Reduce Cyclomatic Complexity
Taking steps to reduce cyclomatic complexity can be beneficial for 3 major reasons:
- Code with high cyclomatic complexity is often more challenging to understand, making it harder for developers to identify and rectify bugs or implement new features.
- Elevated levels of code cyclomatic complexity can lead to decreased performance and increased resource consumption.
- Complex code may be less inclined to thorough testing due to the sheer volume of potential execution paths.
Several strategies exist for reducing cyclomatic complexity within an application’s source code. These approaches aim not only to decrease the number of distinct execution paths but also to simplify decision-making processes within individual functions or methods.
One effective method for reducing cyclomatic complexity is breaking down large functions into smaller, more focused units responsible for specific tasks. Modularizing code in this manner facilitates easier comprehension and debugging while simultaneously promoting reusability across various components of the application.
Another technique involves adopting clear, concise branching structures that minimize nested statements such as if-else blocks or loops within loops. Essentially, developers can enhance the readability and maintainability of their code without sacrificing functionality by reducing nested structures’ depth in favor of simpler constructs like switch-case statements or early returns.
Programmers can also use design patterns that emphasize separation of concerns and encapsulation to significantly reduce cyclomatic complexity. These patterns ensure that each class or module has a single, well-defined responsibility, minimizing the interdependencies between different parts of the codebase.
Similarly, automated testing tools can effectively manage cyclomatic complexity. These tools allow developers to write test cases for individual functions, classes, or modules, guaranteeing that they function correctly within various scenarios. Rigorous testing can then help identify areas where code could be further simplified or refactored to improve overall maintainability.
Understanding how to reduce cyclomatic complexity is necessary when crafting efficient, maintainable software applications that are simple to comprehend and modify. Cyclomatic complexity analysis serves as an invaluable tool for guiding program optimizations, simplifying future development efforts, and enabling developers to strike the perfect balance between creating powerful applications and effectively managing intricate codebases.